Note
Go to the end to download the full example code.
Morphological operations#
This example shows a series of grey scale and binary moprhological operations
import matplotlib.pyplot as plt
import spam.helpers
import spam.measurements
import spam.filters
import spam.datasets
Read the data from datasets and convert#
im = spam.datasets.loadConcreteNe()
# tifffile is reading images in the z,y,x order
sizeZ = im.shape[2]
sizeY = im.shape[1]
sizeX = im.shape[0]
print('Number of pixels: z={}, y={}, x={}'.format(sizeZ, sizeY, sizeX))
# should be unsigned int 16 (16 bits or 2 bytes)
print('Encoded type before: {} -> max={}, min={}'.format(im[0, 0, 0].dtype, im.max(), im.min()))
# convert to unsigned int 8 (8 bits or 1 byte )
im = spam.helpers.convertUnsignedIntegers(im, nBytes=1)
print('Encoded type after: {} -> max={}, min={}'.format(im[0, 0, 0].dtype, im.max(), im.min()))
plt.figure()
plt.imshow(im[sizeZ // 2, :, :], cmap="Greys")
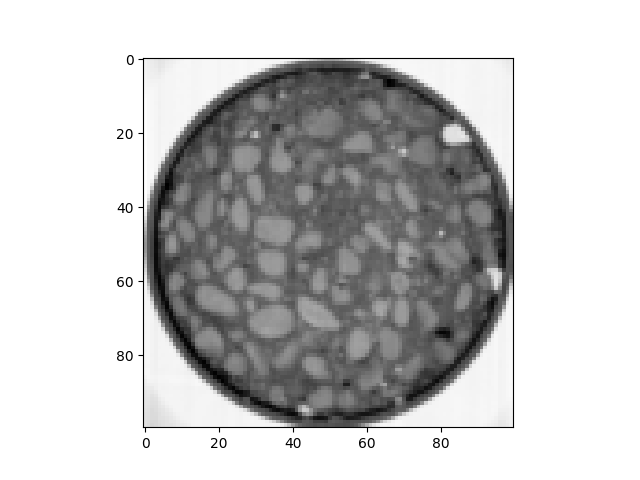
Number of pixels: z=100, y=100, x=100
Encoded type before: uint16 -> max=65535, min=2866
Encoded type after: uint8 -> max=255, min=11
<matplotlib.image.AxesImage object at 0x7f11a7fbd1b0>
Grey scale morphological operations#
We start with o greyscale erosion
tmp = spam.filters.greyErosion(im)
plt.figure()
plt.imshow(tmp[sizeZ // 2, :, :], cmap="Greys")
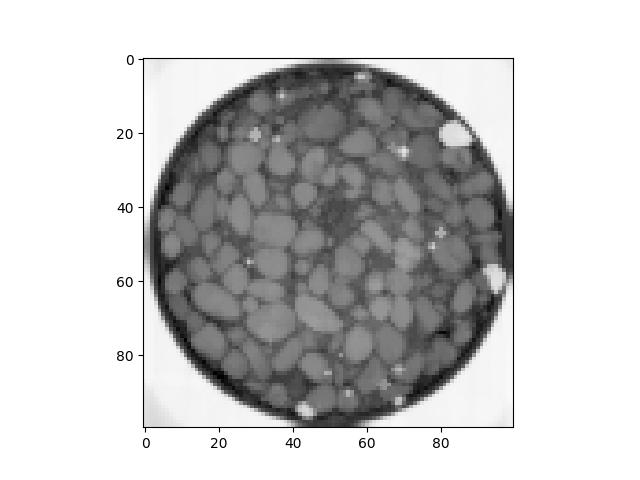
<matplotlib.image.AxesImage object at 0x7f11a7fbd8d0>
And then apply a morphologcial gradient
greyGrad = spam.filters.greyMorphologicalGradient(tmp)
plt.figure()
plt.imshow(greyGrad[sizeZ // 2, :, :])
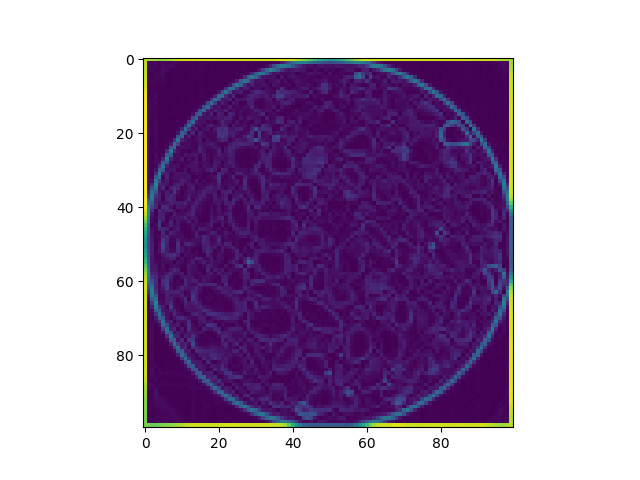
<matplotlib.image.AxesImage object at 0x7f11a751f190>
Threshold the image in order to have binary morphology#
With a threshold of 150 it only keeps the mortar matrix
binIm = im > 150.0 # i.e., binary is where im is bigger than 150
plt.figure()
plt.imshow(binIm[sizeZ // 2, :, :], cmap="Greys")
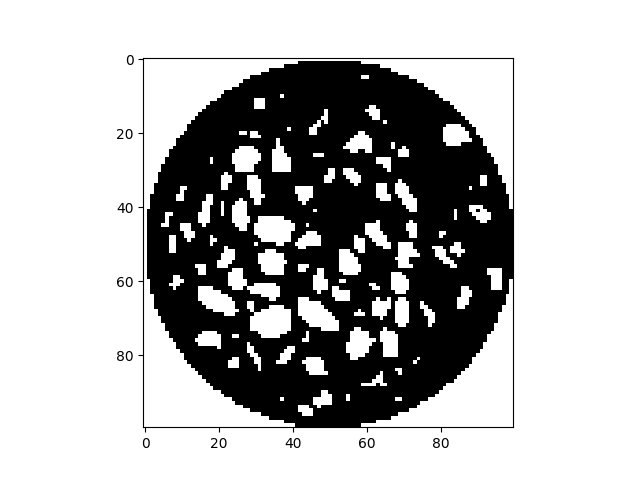
<matplotlib.image.AxesImage object at 0x7f11a784baf0>
Binary morphological operations#
We start with an erosion of the thresholded image
tmp = spam.filters.binaryErosion(binIm)
plt.figure()
plt.imshow(tmp[sizeZ // 2, :, :], cmap="Greys")
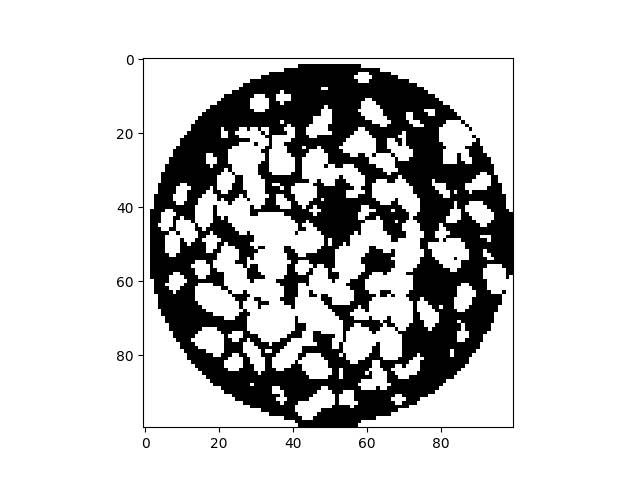
<matplotlib.image.AxesImage object at 0x7f11a7bd7430>
And then apply a morphologcial gradient
binGrad = spam.filters.binaryMorphologicalGradient(tmp)
plt.figure()
plt.imshow(binGrad[sizeZ // 2, :, :], cmap="Greys")
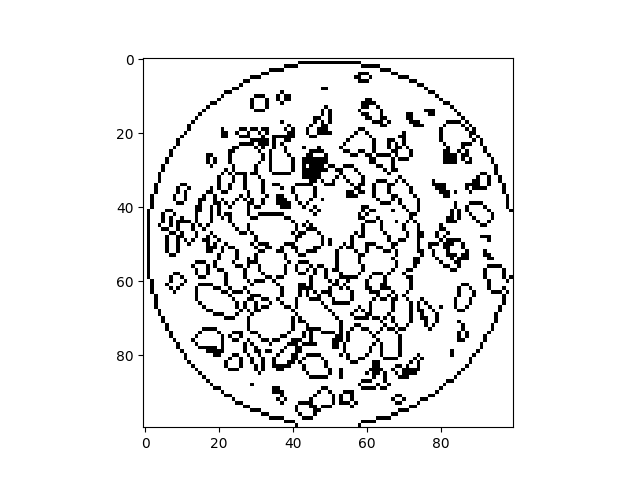
<matplotlib.image.AxesImage object at 0x7f11a7bed0c0>
Compute global descriptors#
print("Measure 0 is the euler characteristic: {:.0f} ".format(spam.measurements.generic(binIm, 0)))
print("Measure 2 is the surface area : {:0.4f}".format(spam.measurements.generic(binIm, 2)))
print("Measure 3 is the volume fraction : {:0.4f}".format(spam.measurements.generic(binIm, 3)))
plt.show()
Measure 0 is the euler characteristic: 660
Measure 2 is the surface area : 167082.1094
Measure 3 is the volume fraction : 585758.0000
Total running time of the script: (0 minutes 0.515 seconds)